What is a bookmarklet
A bookmarklet is simply a piece of Javascript code that can be embedded in your web browser’s bookmark bar. This Javascript code can run in the context of the web page that you are currently viewing in your browser tab, and then perform a variety of tasks on the page for you. As to what a bookmarklet can do, it is only up to your imagination and creativity.
In this post, we’ll explore step-by-step on how to make a simple article reader bookmarklet that generates a clutter-free, simplified view of any web page, making it easy on the eyes. We’ll also look at how to set up the bookmarklet on your web browser. This tutorial assumes you have basic knowledge of HTML, CSS and Javascript. Also, we’ll be using Mozilla’s Readability library for extracting web page content for our bookmarklet.
Let’s start!
Step 1 : Sizing up the article reader bookmarklet
Before we start to code, we’ll want to have an idea of:
- What we want the bookmarklet to do
- How the user interface (UI) would look like
This should be easy because we are going for something really simple. To answer the 2 questions above, we will be building a tool that extracts only the important content sections of any web page and placing them in a side panel for easy viewing and reading. Here’s a screenshot of what the finished article reader bookmarklet would look like:
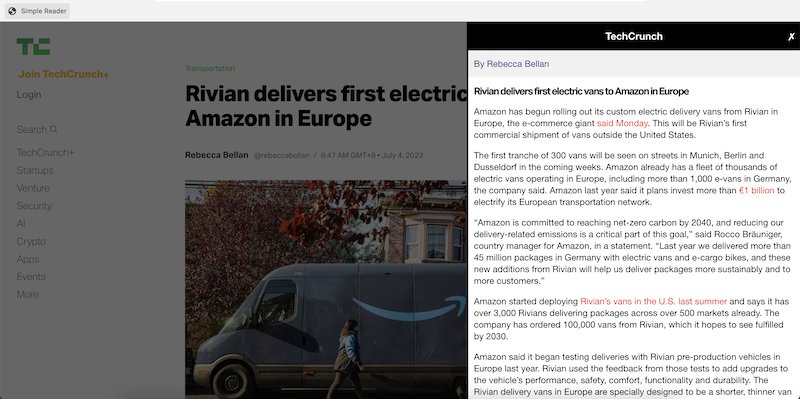
Step 2 : Create a text file for your bookmarklet’s Javascript code
Use your favourite text editor and create a new file. Let’s call it “simple_article_reader.js”. Next, start off your file with this code wrapper:
javascript: (() => { // All Javascript code logic, CSS and HTML here })();
This is the standard “container” for the article reader bookmarklet and all your Javascript code logic, HTML and CSS will subsequently go into this container.
Step 3 : Including the readability Javascript library
We will add the readability library from the JSDelivr CDN to our bookmarklet script. This script will then be injected into the web page’s DOM (in the <head> section) once the bookmarklet is triggered.
javascript: (() => { /* Add the readability library */ let __read = document.createElement('script'); __read.setAttribute("src","https://cdn.jsdelivr.net/npm/moz-readability@0.2.1/Readability.min.js"); let __head = document.head || document.getElementsByTagName('head')[0]; __head.appendChild(__read); .... })();
Notice the double underscore (__) used to declare the Javascript variables? This is to prevent variable name clashes with the main web page’s Javascript. Remember our bookmarklet Javascript will run in the context of the web page we trigger it on.
Step 4 : Writing the CSS for the article reader bookmarklet
In our case, our article reader bookmarklet UI comprises a side panel of 600px in width, is positioned on the right, and anchors to the top and bottom of the page. It has a header that shows the name of the website and a close button to the far right of the header for closing the panel. It also has an author byline and a content panel that is vertically scrollable if the content gets long. With this, we set up the CSS as such:
javascript: (() => { .... let __css = ` body{ overflow-y:hidden; } .panel_mask{ position:fixed; top:0; left:0; right:0; bottom:0; background:#000000; opacity:0.6; z-index:9999999998; } .simple_reader_close{ position:absolute; right:10px; color:#ffffff; } #simple_reader_panel{ width:600px; position:fixed; top:0; bottom:0; right:0px; background:#ffffff; border-left:3px solid #000000; z-index:9999999999; box-sizing:border-box; } #simple_reader_panel .head{ position:relative; font-weight:bold; text-align:center; color:#ffffff; background:#000000; font-size:18px !important; padding:10px; } #simple_reader_panel .author{ font-size:16px !important; padding:10px; color:#333333; width:100%; background:#f1f1f1; } #the_content_window{ width:100%; height:90vh; overflow:auto; padding:10px; padding-bottom:50px; font-size:11px; } #simple_reader_panel p{ font-size:16px !important; line-height:21px !important; color:#000000; margin:10px 0px; } #simple_reader_panel p a{ font-size:16px !important; line-height:19px !important; color:red; } #simple_reader_panel p a:hover{ background:red; text-decoration:none; color:#ffffff; } .a_title{ font-size: 18px; font-weight: bold; margin:10px 0px; } #the_content_window h2{ font-size:14px; } #the_content_window h3{ font-size:13px; } #the_content_window h5{ font-size:12px; } #the_content_window h6{ font-size:12px; } `; let __style = document.createElement('style'); __style.id = "simple_reader_css"; if (__style.styleSheet){ __style.styleSheet.cssText = __css; } else { __style.appendChild(document.createTextNode(__css)); } __head.appendChild(__style); .... })();
Likewise, we will append the CSS style to the <head> of the document too. Next, setting up the Javascript!
Step 5 : Setting up the Javascript
In this last step for our article reader bookmarklet, we will create the side panel UI using HTML and Javascript:
javascript: (() => { .... let __body = document.body || document.getElementsByTagName('body')[0]; window.setTimeout(function(){ let __documentClone = document.cloneNode(true); let __article = new Readability(__documentClone).parse(); let __mask = document.createElement('div'); __mask.classList.add('panel_mask'); __body.appendChild(__mask); let __panel = document.createElement('div'); __panel.id = 'simple_reader_panel'; let __html = '<div class="head">'+__article.siteName; __html += '<a href="javascript:;" class="simple_reader_close" onclick="close_reader_panel()">✗</a>'; __html += '</div>'; __html += '<div class="author"><a href="'+window.location.href+'">'+__article.byline+'</a></div>'; __html += '<div id="the_content_window">'; __html += '<h1 class="a_title">'+__article.title+'</h1>'; __html += __article.content; __html += '</div>'; __panel.innerHTML = __html; __body.appendChild(__panel); },1000); .... })();
Notice a setTimeout function with a 1 second delay? This is to provide a short delay for the readability library to load before our Javascript can function properly.
Finally, we will also add a function for the user to close the bookmarklet panel:
window.close_reader_panel = function(){ document.querySelector('#simple_reader_css').remove(); document.querySelector('#simple_reader_panel').remove(); };
The full code for the bookmarklet would look like this:
javascript: (() => { let __read = document.createElement('script'); __read.setAttribute("src","https://cdn.jsdelivr.net/npm/moz-readability@0.2.1/Readability.min.js"); let __head = document.head || document.getElementsByTagName('head')[0]; __head.appendChild(__read); let __css = ` body{ overflow-y:hidden; } .panel_mask{ position:fixed; top:0; left:0; right:0; bottom:0; background:#000000; opacity:0.6; z-index:9999999998; } .simple_reader_close{ position:absolute; right:10px; color:#ffffff; } #simple_reader_panel{ width:600px; position:fixed; top:0; bottom:0; right:0px; transition:0.5s right; background:#ffffff; border-left:3px solid #000000; z-index:9999999999; box-sizing:border-box; } #simple_reader_panel .head{ position:relative; font-weight:bold; text-align:center; color:#ffffff; background:#000000; font-size:18px !important; padding:10px; } #simple_reader_panel .author{ font-size:16px !important; padding:10px; color:#333333; width:100%; background:#f1f1f1; } #the_content_window{ width:100%; height:90vh; overflow:auto; padding:10px; padding-bottom:50px; font-size:11px; } #simple_reader_panel p{ font-size:16px !important; line-height:21px !important; color:#000000; margin:10px 0px; } #simple_reader_panel p a{ font-size:16px !important; line-height:19px !important; color:red; } #simple_reader_panel p a:hover{ background:red; text-decoration:none; color:#ffffff; } .a_title{ font-size: 18px; font-weight: bold; margin:10px 0px; } #the_content_window h2{ font-size:14px; } #the_content_window h3{ font-size:13px; } #the_content_window h5{ font-size:12px; } #the_content_window h6{ font-size:12px; } `; let __style = document.createElement('style'); __style.id = "simple_reader_css"; if (__style.styleSheet){ __style.styleSheet.cssText = __css; } else { __style.appendChild(document.createTextNode(__css)); } __head.appendChild(__style); let __body = document.body || document.getElementsByTagName('body')[0]; window.setTimeout(function(){ let __documentClone = document.cloneNode(true); let __article = new Readability(__documentClone).parse(); let __mask = document.createElement('div'); __mask.classList.add('panel_mask'); __body.appendChild(__mask); let __panel = document.createElement('div'); __panel.id = 'simple_reader_panel'; let __html = '<div class="head">'+__article.siteName; __html += '<a href="javascript:;" class="simple_reader_close" onclick="close_reader_panel()">✗</a>'; __html += '</div>'; __html += '<div class="author"><a href="'+window.location.href+'">'+__article.byline+'</a></div>'; __html += '<div id="the_content_window">'; __html += '<h1 class="a_title">'+__article.title+'</h1>'; __html += __article.content; __html += '</div>'; __panel.innerHTML = __html; __body.appendChild(__panel); },1000); window.close_reader_panel = function(){ document.querySelector('#simple_reader_css').remove(); document.querySelector('#simple_reader_panel').remove(); }; })();
Next, we’ll look at how to install and use the bookmarklet!
Step 7 : Install the bookmarklet
First, you need to enable your web browser’s bookmark bar. This can be found in the settings section of the browser you are using. You can also check this article to learn how to turn on your bookmarks bar.
To install the article reader bookmarklet, simply follow the same steps as you would when you create a standard bookmark. Here’s an example for the Brave browser : Right-click on the bookmarks bar and select “Add Page”.
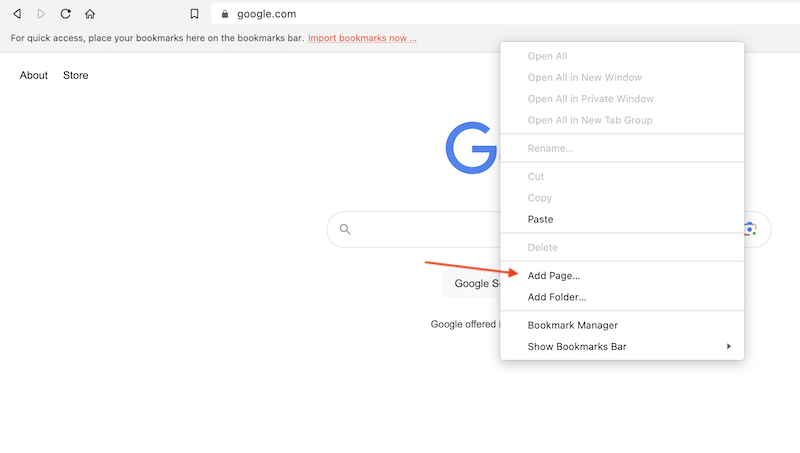
Next, enter “Simple Reader” (or any name you would prefer) in the “Name” field. Then, copy the full code of the bookmarklet and paste it in the “URL” field. Click “Save”. Make sure this is shown on the bookmarks bar so that you can access it conveniently. If everything goes well, you should see the “Simple Reader” on your bookmarks bar.
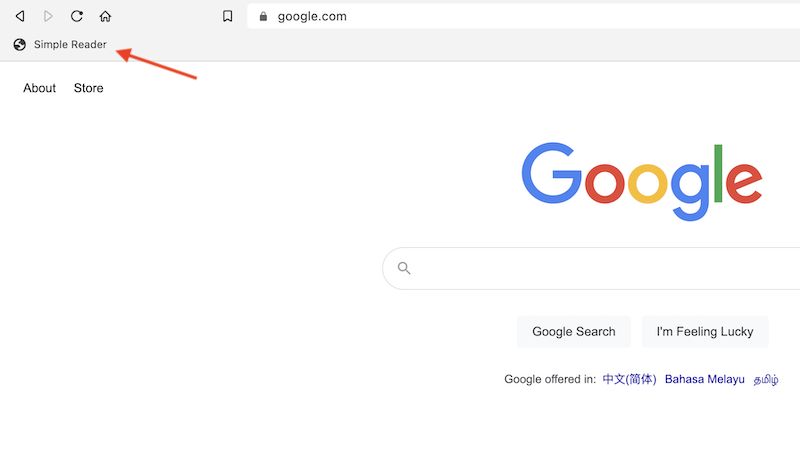
Great. Installation is done! Now, let’s try it out.
Step 8 : Test the Bookmarklet
Visit any web article page. Try this one – https://www.makeuseof.com/chrome-simulate-color-blindness/
Once the page loads, click on your bookmarklet. You will a side panel with the main article sections displayed in a simplified and clutter-free manner.
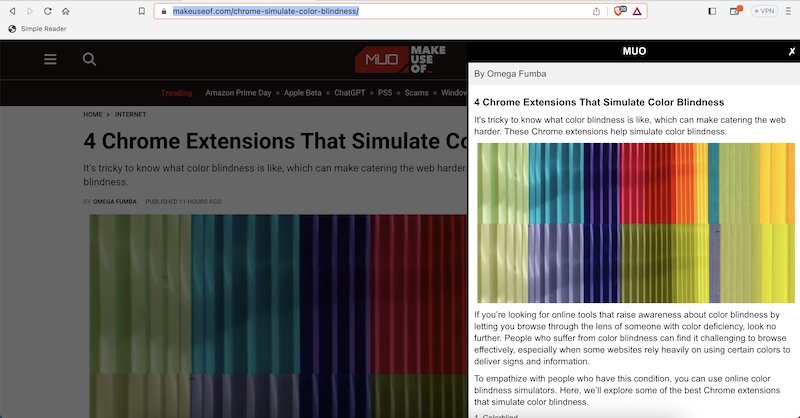
That’s all!